Introduction:
Traditionally, SharePoint custom applications are created using SPFx in SharePoint 2016 and 2019. However, this needs additional server configuration and the react version/SPFx version is also very old.
Today, we can easily use ReactJS to create single page custom applications both for on-prem and online SharePoint covering 2013,2016 and 2019. Although, currently it applies only for SPO classic sites, the ease of development and deployment makes the choice of ReactJS very popular.
The effort here is to describe a step by step approach to create a custom application using latest ReactJS and PnPJS. Hope this will serve as a quick reference to the developers interested in building custom app in SPO.
Project Configuration
- Create a new React App using the following command
npx create-react-app my-app cd my-app
- Open the project in VSCode or WebStorm or any other editor
- Open the terminal
- Run the below command
npm install concurrently sp-rest-proxy –-save-dev
- A successful installation can be confirmed when the below shows up in package.json
- The following command can be run to use pnp js and bootstrap
npm install @pnp/sp react-bootstrap
- Create “api-server.js” file at the root of the solution where package.json file resides
- Open “api-server.js” file and add the below code block
const RestProxy = require('sp-rest-proxy'); const settings = { configPath: './config/private.json', // Location for SharePoint instance mapping and credentials port: 8081, // Local server port //staticRoot: 'node_modules/sp-rest-proxy/static', // Root folder for static content }; const restProxy = new RestProxy(settings); restProxy.serve();
-
Add the below lines under the package.json scripts block
"proxy": "node ./api-server.js", "startServers": "concurrently --kill-others \"npm run proxy\" \"npm run start\""
-
Confirm that the package.json scripts block appears ike the one below
"scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject", "proxy": "node ./api-server.js", "startServers": "concurrently --kill-others \"npm run proxy\" \"npm run start\"" }
-
Add below code block to the end of package.json
"proxy": "http://127.0.0.1:8081"
Successful implementation of all the above changes can be confirmed when packge.json file appears like the one below
{
"name": "sp-react-app",
"version": "0.1.0",
"private": true,
"dependencies": {
"@pnp/sp": "^2.0.5",
"@testing-library/jest-dom": "^4.2.4",
"@testing-library/react": "^9.5.0",
"@testing-library/user-event": "^7.2.1",
"bootstrap": "^4.4.1",
"react": "^16.13.1",
"react-bootstrap": "^1.0.1",
"react-dom": "^16.13.1",
"react-scripts": "3.4.1"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"proxy": "node ./api-server.js",
"startServers": "concurrently --kill-others \"npm run proxy\" \"npm run start\""
},
"eslintConfig": {
"extends": "react-app"
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
"devDependencies": {
"concurrently": "^5.2.0",
"sp-rest-proxy": "^2.11.1"
},
"proxy": "http://127.0.0.1:8081",
}
Setup connection to SharePoint
Open terminal to project folder and run the below command.
npm run proxy
Provide the SharePoint site URL, username and password when prompted to start the proxy at localhost:8081. Open http://localhost:8081 in browser and validate that the rest API page can be seen.
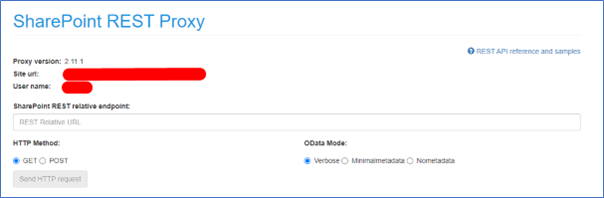
A rest url can also be provided to to validate the connection. “_api/web”
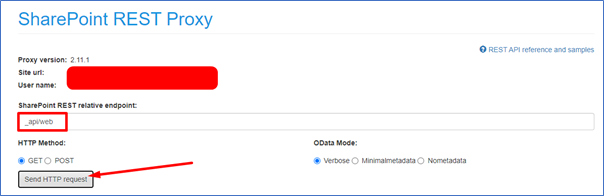
Setup @Pnp/Sp
Open App.js file and add the below constructor.
constructor(props) {
super(props);
sp.setup({
sp: {
headers: {
Accept: 'application/json;odata=verbose',
},
baseUrl: 'http://localhost:3000/',
},
});
}
Connect to SharePoint from Application
Create a new method to get current user information in App.js file.
getCurrentUser = () => {
sp.web.currentUser.get().then((currentUser) => {
console.log('currentUser', currentUser);
});
};
Call the above method in componentDidMount().
Run the project
Open terminal in and run below command.
npm run startServers
Open console of http://localhost:3000 and to see current user information from SharePoint.
Takeaways
We can use this concept to create any custom application in SharePoint where particularly we don’t have an option to create a SPFx solution and want to use ReactJS or any other latest JavaScript framework.
Feel free to comment of get in touch in case you need any clarifications or further help.
Hi,
Thank you for sharing this great article.
I have a scenario I’ll appreciate if you can advise here.
I have already setup on prem sharepoint server. Now I need a stand alone react application which will use sharepoint authentication and get and upload documents to sharepoint.
Any handy code sample would be really a great help.
Thank you
Hai,
It is working for localhost. But when i go to actual SharePoint site it is not working. How can I connect it to the SharePoint server.
error :
Compiled with problems:
×
ERROR
[eslint]
src\App.js
Line 7:2: ‘sp’ is not defined no-undef
Line 20:4: ‘getCurrentUser’ is not defined no-undef
Line 23:5: ‘sp’ is not defined no-undef
Search for the keywords to learn more about each error.